Which of the Following Is a Difference Between the Read and Write Functions?
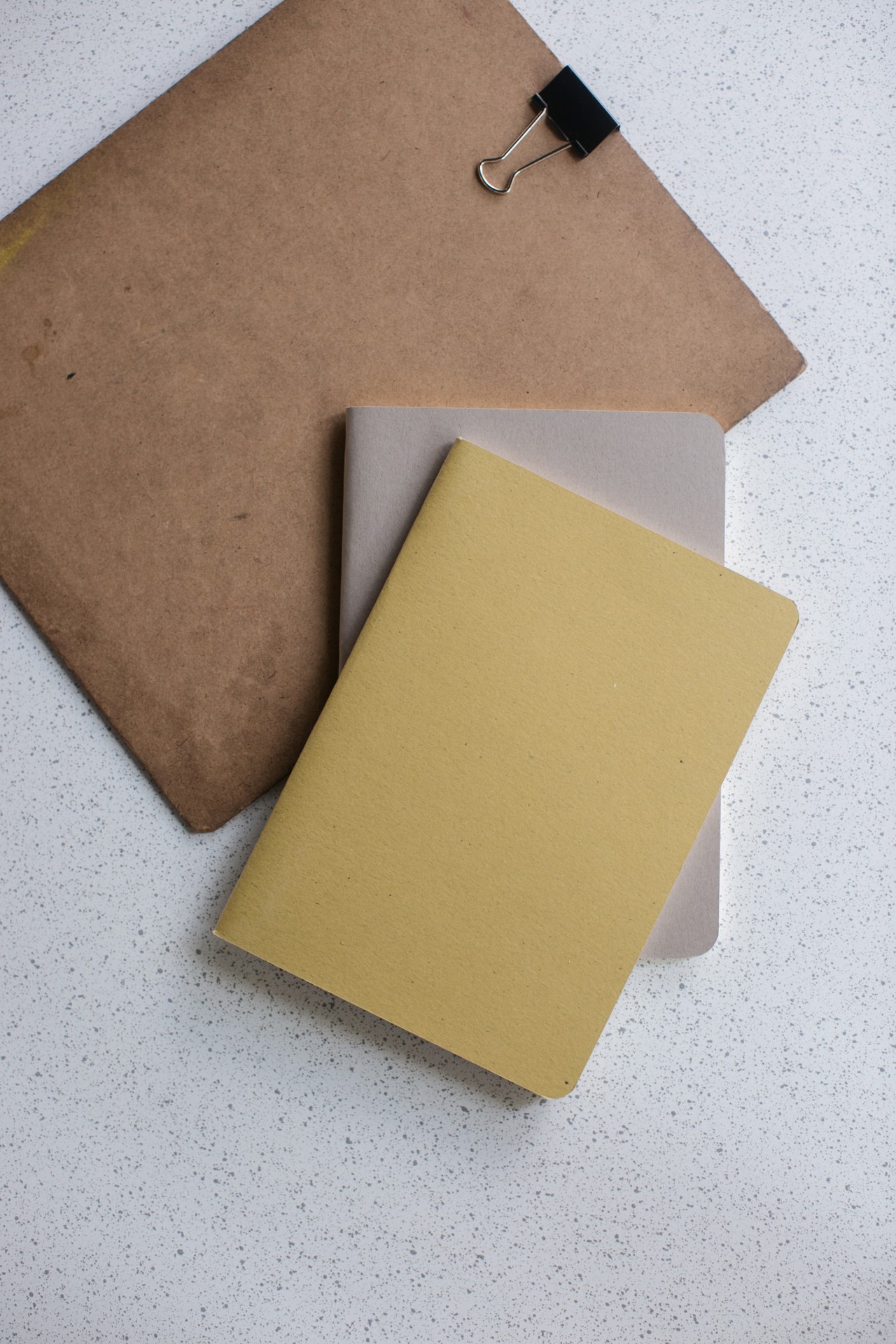
If you've written the C helloworld
program before, y'all already know basic file I/O in C:
/* A uncomplicated hi earth in C. */ #include <stdlib.h> // Import IO functions. #include <stdio.h> int primary() { // This printf is where all the file IO magic happens! // How heady! printf("How-do-you-do, globe!\n"); render EXIT_SUCCESS; }
File handling is ane of the nearly of import parts of programming. In C, nosotros apply a structure arrow of a file blazon to declare a file:
FILE *fp;
C provides a number of build-in part to perform basic file operations:
-
fopen()
- create a new file or open a existing file -
fclose()
- close a file -
getc()
- reads a character from a file -
putc()
- writes a grapheme to a file -
fscanf()
- reads a ready of information from a file -
fprintf()
- writes a set of data to a file -
getw()
- reads a integer from a file -
putw()
- writes a integer to a file -
fseek()
- set the position to desire point -
ftell()
- gives current position in the file -
rewind()
- fix the position to the showtime signal
Opening a file
The fopen()
role is used to create a file or open up an existing file:
fp = fopen(const char filename,const char fashion);
There are many modes for opening a file:
-
r
- open up a file in read fashion -
w
- opens or create a text file in write way -
a
- opens a file in append mode -
r+
- opens a file in both read and write fashion -
a+
- opens a file in both read and write mode -
westward+
- opens a file in both read and write mode
Hither's an case of reading data from a file and writing to it:
#include<stdio.h> #include<conio.h> main() { FILE *fp; char ch; fp = fopen("how-do-you-do.txt", "w"); printf("Enter data"); while( (ch = getchar()) != EOF) { putc(ch,fp); } fclose(fp); fp = fopen("hello.txt", "r"); while( (ch = getc(fp)! = EOF) printf("%c",ch); fclose(fp); }
Now you might be thinking, "This just prints text to the screen. How is this file IO?"
The respond isn't obvious at first, and needs some agreement about the UNIX organisation. In a UNIX organization, everything is treated equally a file, pregnant you can read from and write to it.
This ways that your printer can exist abstracted as a file since all you do with a printer is write with it. It is also useful to think of these files as streams, since as you lot'll see later, y'all can redirect them with the crush.
So how does this relate to helloworld
and file IO?
When you lot call printf
, y'all are really just writing to a special file called stdout
, brusque for standard output . stdout
represents the standard output as decided by your trounce, which is usually the last. This explains why it printed to your screen.
There are two other streams (i.east. files) that are available to you with try, stdin
and stderr
. stdin
represents the standard input , which your crush ordinarily attaches to the keyboard. stderr
represents the standard mistake output, which your shell usually attaches to the terminal.
Rudimentary File IO, or How I Learned to Lay Pipes
Enough theory, let's get down to business by writing some code! The easiest way to write to a file is to redirect the output stream using the output redirect tool, >
.
If you want to append, you lot can use >>
:
# This will output to the screen... ./helloworld # ...only this will write to a file! ./helloworld > hello.txt
The contents of hullo.txt
will, not surprisingly, be
Hello, world!
Say we have another program chosen greet
, similar to helloworld
, that greets you lot with a given name
:
#include <stdio.h> #include <stdlib.h> int main() { // Initialize an array to hold the proper name. char name[twenty]; // Read a cord and save it to name. scanf("%s", name); // Print the greeting. printf("Hullo, %s!", name); return EXIT_SUCCESS; }
Instead of reading from the keyboard, we can redirect stdin
to read from a file using the <
tool:
# Write a file containing a proper name. echo Kamala > proper noun.txt # This will read the name from the file and print out the greeting to the screen. ./greet < proper noun.txt # ==> Hello, Kamala! # If you wanted to too write the greeting to a file, you lot could practise and then using ">".
Notation: these redirection operators are in bash
and similar shells.
The Real Deal
The above methods but worked for the nearly basic of cases. If you wanted to do bigger and ameliorate things, you will probably want to work with files from within C instead of through the shell.
To accomplish this, you will use a function chosen fopen
. This role takes ii string parameters, the first beingness the file proper noun and the 2d being the mode.
The mode are basically permissions, then r
for read, w
for write, a
for append. Yous can likewise combine them, then rw
would mean you could read and write to the file. There are more modes, only these are the most commonly used.
After you have a FILE
arrow, you tin can utilise basically the same IO commands you would've used, except that you have to prefix them with f
and the get-go argument volition be the file pointer. For example, printf
's file version is fprintf
.
Here'due south a program called greetings
that reads a from a file containing a list of names and write the greetings to another file:
#include <stdio.h> #include <stdlib.h> int main() { // Create file pointers. FILE *names = fopen("names.txt", "r"); FILE *greet = fopen("greet.txt", "w"); // Bank check that everything is OK. if (!names || !greet) { fprintf(stderr, "File opening failed!\n"); render EXIT_FAILURE; } // Greetings time! char proper name[xx]; // Basically go on on reading untill there's nothing left. while (fscanf(names, "%s\n", name) > 0) { fprintf(greet, "Hello, %south!\n", name); } // When reached the end, impress a message to the concluding to inform the user. if (feof(names)) { printf("Greetings are done!\north"); } return EXIT_SUCCESS; }
Suppose names.txt
contains the following:
Kamala Logan Carol
And then after running greetings
the file greet.txt
volition contain:
Hello, Kamala! Hello, Logan! Hello, Carol!
Learn to code for gratuitous. freeCodeCamp's open source curriculum has helped more than than xl,000 people go jobs as developers. Get started
downingdrigat1992.blogspot.com
Source: https://www.freecodecamp.org/news/file-handling-in-c-how-to-open-close-and-write-to-files/
0 Response to "Which of the Following Is a Difference Between the Read and Write Functions?"
Post a Comment